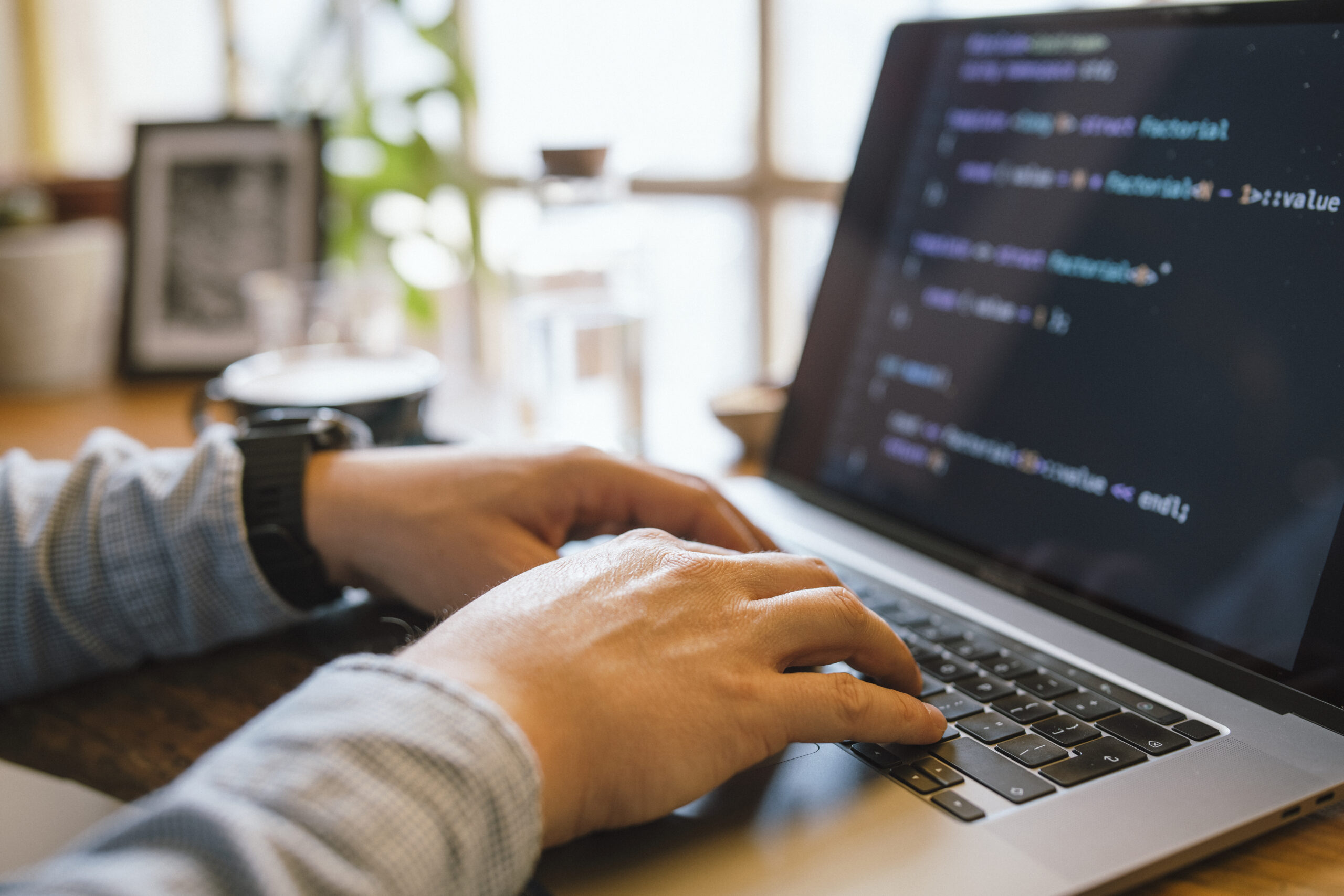
Debugging is The most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to unravel challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your efficiency. Here's many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of advancement, understanding how to connect with it properly in the course of execution is Similarly significant. Modern day improvement environments occur Outfitted with potent debugging abilities — but several builders only scratch the floor of what these resources can perform.
Get, for example, an Built-in Progress Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications help you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and perhaps modify code about the fly. When employed correctly, they Permit you to observe exactly how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of community requests, check out serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around running processes and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default settings and shortcuts — it’s about building an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you already know your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — techniques in productive debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders want to create a consistent ecosystem or circumstance the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, generally resulting in squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, take into consideration creating automatic checks that replicate the edge scenarios or state transitions concerned. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem can be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It needs endurance, observation, and also a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools more successfully, check possible fixes securely, and communicate much more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Browse and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as discouraging interruptions, builders must discover to treat mistake messages as direct communications in the system. They normally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the very first line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the accurate root induce. Don’t just copy and paste mistake messages into serps — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These questions can tutorial your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the mistake happened. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When made use of effectively, it offers serious-time insights into how an software behaves, encouraging you understand what’s going on underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Frequent logging amounts consist of DEBUG, Information, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout growth, Data for basic occasions (like successful get started-ups), Alert for prospective problems that don’t break the applying, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Target crucial events, condition adjustments, enter/output values, and demanding decision details inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-believed-out logging tactic, you are able to decrease the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working advanced problems into manageable elements and comply with clues logically to uncover the basis bring about.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece jointly a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What could be causing this actions? Have any improvements not long ago been manufactured to your codebase? Has this issue transpired ahead of below comparable circumstances? The target is usually to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled environment. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you nearer to the truth.
Pay near interest to smaller specifics. Bugs often conceal in the minimum expected spots—like a missing semicolon, an off-by-a person error, or simply a race issue. Be complete and individual, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the real dilemma, just for it to resurface later.
And finally, continue to keep notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and grow to be simpler at uncovering concealed issues in complicated programs.
Produce Checks
Writing exams is one of the best solutions to help your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but will also function a security Web that offers you confidence when creating adjustments in your codebase. A properly-examined application is simpler to debug since it permits you to pinpoint specifically the place and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where by to glance, appreciably minimizing time invested debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand currently being set.
Next, combine integration exams and finish-to-end assessments into your workflow. These aid make sure that many portions of your application do the job jointly easily. They’re significantly handy for catching bugs that take place in complex devices with several factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This level of knowledge Normally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. When the test fails constantly, you may concentrate on repairing the bug and check out your test move when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing recreation right into a structured and predictable system—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the situation—gazing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging instruments is solely stepping absent. Getting breaks can help you reset your head, cut down irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could commence overlooking clear problems or misreading code which you wrote just hours earlier. In this point out, your Mind gets significantly less efficient at problem-resolving. A brief stroll, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate within the background.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent permits you to read more return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
If you’re caught, a great guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is part of fixing it.
Master From Every Bug
Every single bug you come upon is more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you anything important if you make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you figured out. After some time, you’ll begin to see patterns—recurring issues or popular issues—you can proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the similar concern boosts team effectiveness and cultivates a more powerful Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary parts of your progress journey. In any case, a lot of the ideal builders usually are not those who create great code, but people who repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive away a smarter, extra capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.